现实的例子
现实生活中适配器的例子,比如笔记本电脑的电源适配器,可以将 220V 电压转换为笔记本电脑需要的 12V 电压。在软件设计模式中,适配器模式也是类似的,它可以将一个类的接口转换为另一个类的接口,让原本由于接口不兼容而不能一起工作的类可以一起工作。
适配器模式解决什么问题
接口不兼容问题:当一个类的接口与另一个类的接口不兼容时,可以使用适配器模式将一个类的接口转换为另一个类的接口。比如新开发的系统要接入旧系统的某些功能时,就可以使用适配器模式。
示例讲解
有一个类Target
,它有一个operation
方法,但是现在需要将使用一个类Adaptee
的specificOperation
方法。此时可以借助Adapter
模式,我们创建一个类Adapter
,继承Target
类,并持有一个Adaptee
类的实例。在Adapter
类的operation
方法中,调用Adaptee
的specificOperation
方法。
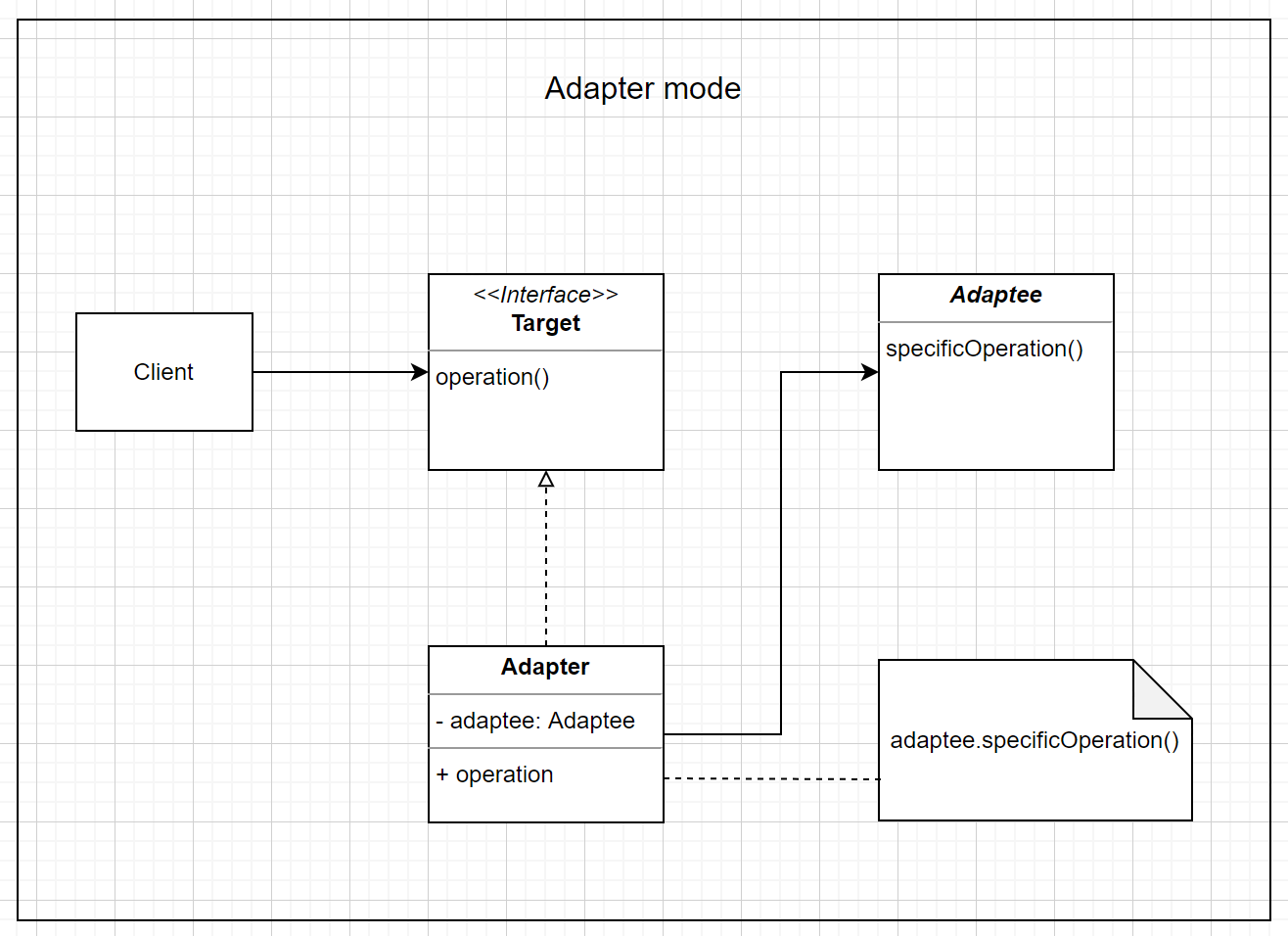
完整代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| class Target { public operation() { console.log(`Target: The default operation.`); } }
class Adaptee { public specificOperation() { console.log("Adaptee: Specific operation."); } }
class Adapter extends Target { private adaptee: Adaptee;
constructor(adaptee: Adaptee) { super(); this.adaptee = adaptee; }
public operation() { this.adaptee.specificOperation(); } }
function clientCode(target: Target) { target.operation(); }
const target = new Target(); clientCode(target);
const adaptee = new Adaptee(); const adapter = new Adapter(adaptee); clientCode(adapter);
|